반응형
Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 자바스크립트
- Vue
- mapGetters
- 댓글달기
- ES6
- v-html
- vuex
- State
- nodejs
- jsx
- storybook
- HOC
- App.vue
- express
- TypeScript
- 자료구조
- MySQL
- input
- event
- Vue transition
- 쉬운설명
- react
- 리액트
- sass
- webpack
- scss
- JavaScript
- Vue.js
- CSS
- Wecode
Archives
- Today
- Total
익명의 개발노트
[Linear] Doubly linked list 본문
반응형
doubly linked list는 양방향 링크드 리스트라고 불리운다.
양방향이기 때문에 단방향 리스트하고 비교시 이전값을 가리키는 포인터가 들어있다.
1. Linked List와 차이점
1) 시작과 끝 부분이 거꾸로도 가능하다는 의미이다.
2) 원하는 element의 이전값이나 다음값으로도 갈 수 가 있다.
3) 단방향에서는 원하는 element를 놓쳤을 경우 처음부터 돌아가서 탐색해야하는데, 양방향은 그럴 필요없다.
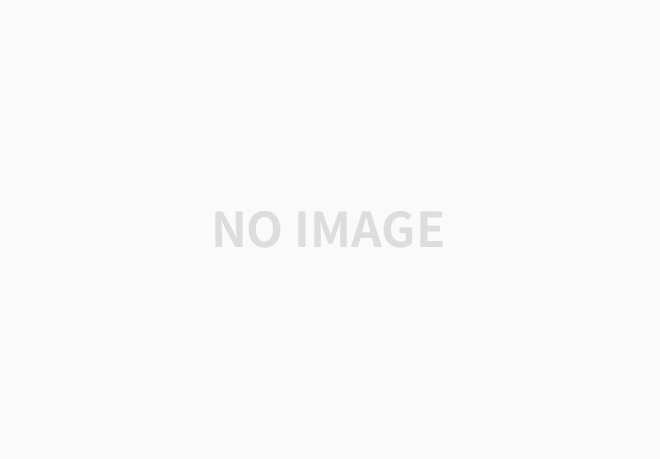
2.functional instantiation
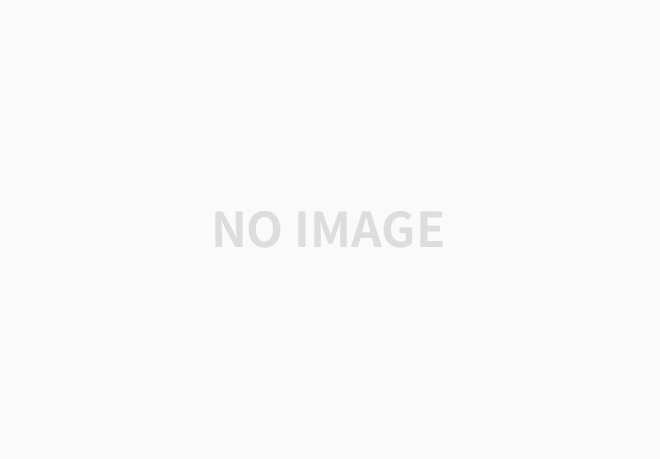
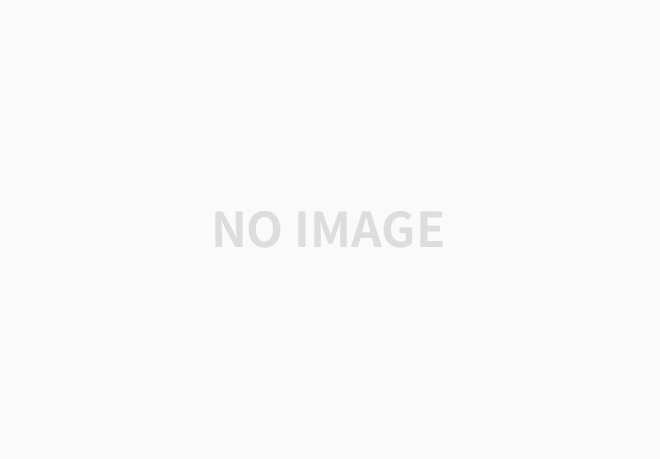
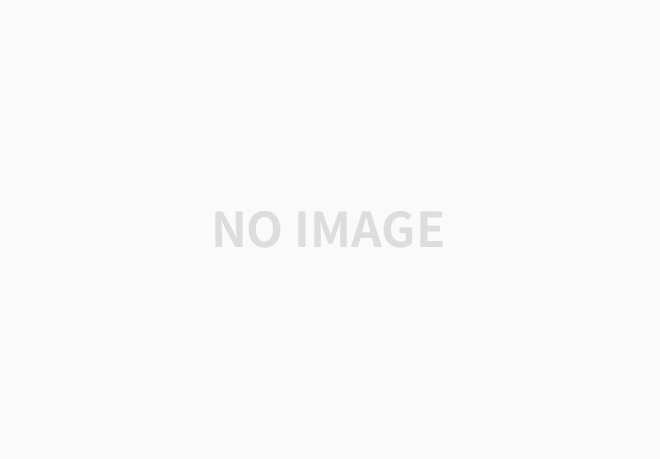
function DoublyLinkedList(){
let Node = function(item){
this.element = item;
this.next = null;
this.prev = null; //추가됨.
};
let length = 0;
let head = null;
let tail = null; //추가됨.
this.insert = function (position, item){
if(position >= 0 && position <= length){
let node = new Node(item);
let current = head; // current는 현재 있는 노드들이야 그냥.
let previous; //추가됨.
let index =0;
if(position === 0){ //처음자리에 추가.
if(!head){ //추가된 부분.
head = node;
tail = node;
}else{
node.next = current;
current.previous = node; //추가된 부분
head = node;
}
}else if(position === length){ //추가된 부분, 마지막 item찾기. 마지막부분에 추가.
current = tail;
current.next = node;
node.prev = current;
tail = node;
}else{ //중간에 넣기
while(index++ < position){
previous = current;
current = current.next;
}
node.next = current; //추가된 부분
previous.next = node; // 추가된 부분
}
length++;
return true;
}else{
return false;
}
}//end insert
this.removeAt = function(position){
if(position > -1 && position < length){
let current = head;
let previous;
let index =0;
if(postion === 0){ //첫번째 항목제거.
head = current.next;
//추가된 부분임. 만약 리스트 전체길이가 1인경우 tail을 업데이트해야한다.
if(length ===1){
tail = null
}else{
head.prev = null;
}
}else if(position === length-1){ //추가된 부분,마지막 아이템 제거
current = tail;
tail = current.prev;
tail.next = null;
}else{ //중간값 제거.
while(index++ < position){
previous = current;
current = current.next;
}
previous.next = current.next;
current.next.prev = previous; //추가된 부분
}
length --;
return current.item;
}else{
return null;
}
}// end removeAt
//나머지는 동일.
}
반응형
'프로그래밍 관련자료 > 자료구조 및 Big-O' 카테고리의 다른 글
[Non-Linear] Hash Table (0) | 2019.04.21 |
---|---|
[Non-Linear] Graph 구조 (0) | 2019.04.15 |
[Non-Linear] Binary - heap (0) | 2019.04.15 |
[Non-Linear] 트리구조 순회방법(Tree traversals) (0) | 2019.04.15 |
[Non-Linear] 트리구조 (0) | 2019.04.15 |